Title: Unraveling the Marvels of Factory Design Patterns
Introduction:
Factory design patterns are a cornerstone in object-oriented programming, providing an elegant solution to create objects without specifying their exact class. In object-oriented programming, they play a crucial role in promoting code flexibility, maintainability, and scalability. Let's delve into the details of factory design patterns and explore them with some examples that showcase their power.
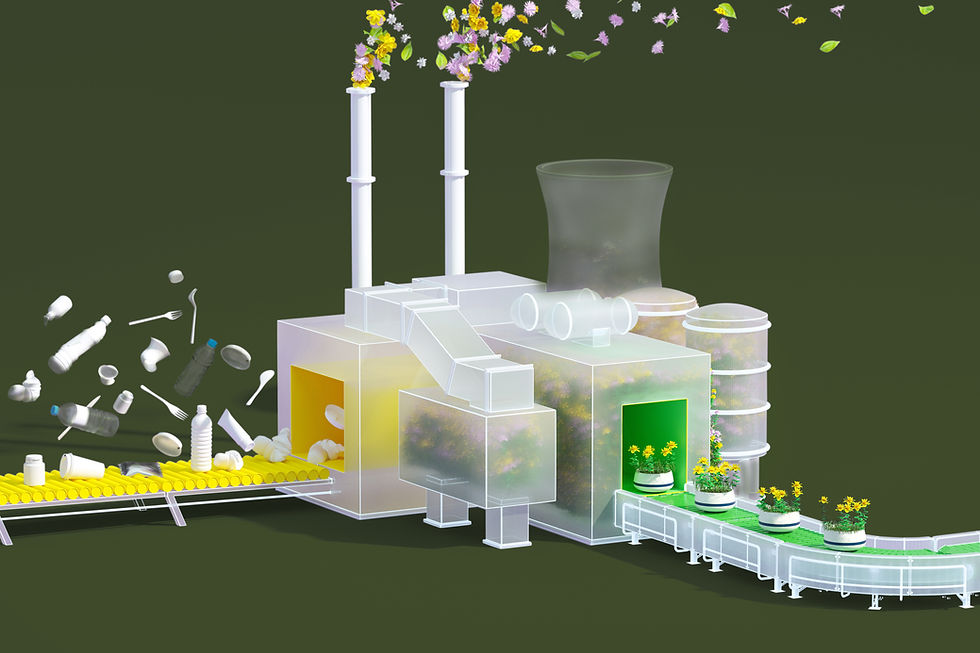
The Basics of Factory Design Patterns:
At its core, the factory pattern involves creating objects without specifying the exact class of the object that will be created. This is achieved by defining an interface for creating an object but letting subclasses alter the type of objects that will be created.
public interface IProduct
{
void DisplayInfo();
}
public class ConcreteProductA : IProduct
{
public void DisplayInfo()
{
Console.WriteLine("This is ConcreteProductA.");
}
}
public class ConcreteProductB : IProduct
{
public void DisplayInfo()
{
Console.WriteLine("This is ConcreteProductB.");
}
}
public interface IFactory
{
IProduct CreateProduct();
}
public class ConcreteFactoryA : IFactory
{
public IProduct CreateProduct()
{
return new ConcreteProductA();
}
}
public class ConcreteFactoryB : IFactory
{
public IProduct CreateProduct()
{
return new ConcreteProductB();
}
}
Dynamic Object Creation:
One fascinating aspect of factory patterns in C# is their ability to dynamically create objects based on runtime conditions. This makes them incredibly versatile in scenarios where the exact type of object needed is determined during program execution.
public class DynamicObjectCreator
{
public IProduct CreateProduct(bool condition)
{
return condition
? (IProduct)new ConcreteFactoryA()
.CreateProduct()
: (IProduct)new ConcreteFactoryB()
.CreateProduct();
}
}
Usage:
var creator = new DynamicObjectCreator();
var product = creator.CreateProduct(true);
product.DisplayInfo(); // Output: "This is ConcreteProductA.
Singleton Factory
Combining the factory pattern with the singleton pattern can lead to efficient and resource-friendly implementations. This ensures that a single instance of a factory is responsible for creating objects throughout the application.
public class SingletonFactory
{
private static readonly IFactory factoryInstance = new ConcreteFactoryA();
private SingletonFactory() { }
public static IFactory FactoryInstance => factoryInstance;
public IProduct CreateProduct()
{
return factoryInstance.CreateProduct();
}
}
Usage:
var singletonFactory = SingletonFactory.FactoryInstance;
var productFromSingleton = singletonFactory.CreateProduct();
productFromSingleton.DisplayInfo(); // Output: "This is ConcreteProductA."
Generic Factory:
C# supports generics, and leveraging them in factory patterns can lead to more flexible and reusable code. A generic factory allows you to create objects of various types without sacrificing type safety.
public class GenericFactory<TProduct> where TProduct : IProduct, new()
{
public TProduct CreateProduct()
{
return new TProduct();
}
}
Usage:
var genericFactory = new GenericFactory<ConcreteProductB>();
var productFromGeneric = genericFactory.CreateProduct();
productFromGeneric.DisplayInfo(); // Output: "This is ConcreteProductB."
Conclusion:
Factory design patterns in C# in the case above provide an elegant way to create objects with flexibility and extensibility. Whether it's dynamically creating objects, implementing singletons, or harnessing the power of generics, the factory pattern proves to be a versatile tool in a C# developer's arsenal. By understanding and applying these concepts, you can enhance your code's maintainability and scalability while promoting good design practices.
😊
Nice article