Why is the Liskov Substitution Principle Important for Object-Oriented Programming
- amol ankit
- Nov 9, 2023
- 3 min read
Title: Mastering Software Design with the Liskov Substitution Principle (LSP)
Introduction
Effective software design is a crucial aspect of creating robust, maintainable, and scalable software applications. The Liskov Substitution Principle (LSP) is one of the key SOLID design principles, originally introduced by Barbara Liskov. This article will delve into the Liskov Substitution Principle, understand its significance, and explore how it can revolutionize your software design practices.
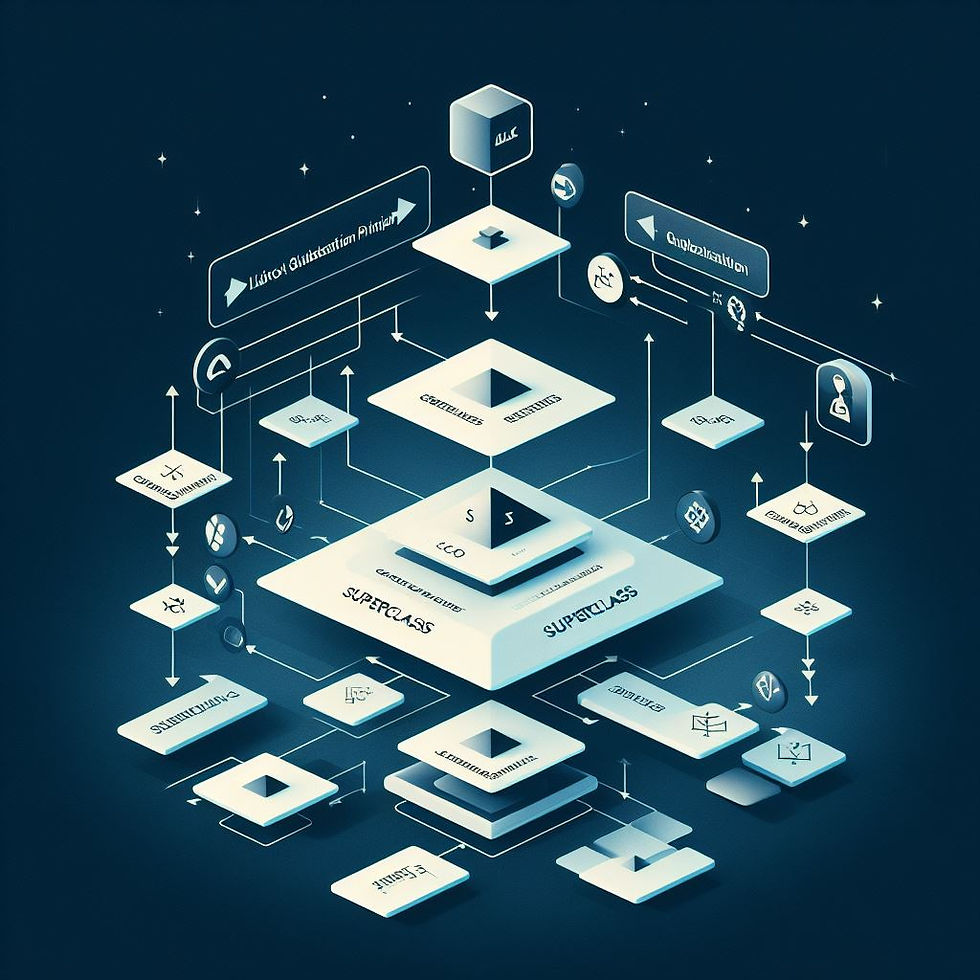
What is the Liskov Substitution Principle (LSP)?
The Liskov Substitution Principle is one of the five SOLID principles of object-oriented design. It can be defined as follows: Subtypes must be substitutable for their base types without altering the correctness of the program. In simpler terms, if a class is a subtype of another class, it should be able to be used interchangeably with its base class without causing issues.
Key Benefits of LSP
Enhancing Code Extensibility: Adhering to the LSP enables you to create new derived classes that can extend the functionality of base classes without breaking the existing code. This promotes easy and safe code extensibility.
Ensuring Consistency: By maintaining the substitutability of derived classes for base classes, you ensure that the behaviour of your code remains consistent. This consistency simplifies the development process and reduces the likelihood of introducing errors.
Facilitating Polymorphism: The LSP is a cornerstone of polymorphism, which allows you to write more generic code that can work with various derived classes without knowing their specific implementations. This promotes code reusability and flexibility.
Reducing Bugs: Ensuring that derived classes can be used seamlessly in place of their base classes helps prevent unexpected bugs and errors. It eliminates the need to inspect and adjust the code every time a new derived class is added.
How to Apply LSP in Your Code
Understand the "Is-A" Relationship: Before creating derived classes, ensure they genuinely inherit the "is-a" relationship with the base class. In other words, the derived class should be a specialized version of the base class.
Preserve Contractual Agreements: Ensure that the derived classes maintain the contractual agreements (preconditions and postconditions) established by the base class. Violating these agreements can lead to unexpected behaviour.
Avoid Overrides That Alter Behavior: Be cautious when overriding methods from the base class. Overridden methods should either preserve the behaviour of the base class or extend it without changing the fundamental functionality.
Perform Rigorous Testing: Thoroughly test your code, especially when working with derived classes. Test the derived classes to ensure they uphold the LSP and can be used interchangeably with base classes.
Real-World Example
Imagine you are working on a geometry library that handles various shapes, such as circles and rectangles. Your base class, "Shape," defines common methods like "calculateArea" and "calculatePerimeter." When you create derived classes like "Circle" and "Rectangle," they should adhere to the Liskov Substitution Principle, allowing you to use them interchangeably with the base class, "Shape," in any context where a "Shape" is expected.
using System;
public abstract class Shape
{
public abstract double CalculateArea();
public abstract double CalculatePerimeter();
}
public class Circle : Shape
{
public double Radius { get; set; }
public Circle(double radius)
{
Radius = radius;
}
public override double CalculateArea()
{
return Math.PI * Math.Pow(Radius, 2);
}
public override double CalculatePerimeter()
{
return 2 * Math.PI * Radius;
}
}
public class Rectangle : Shape
{
public double Width { get; set; }
public double Height { get; set; }
public Rectangle(double width, double height)
{
Width = width;
Height = height;
}
public override double CalculateArea()
{
return Width * Height;
}
public override double CalculatePerimeter()
{
return 2 * (Width + Height);
}
}
public class Program
{
public static void PrintShapeDetails(Shape shape)
{
Console.WriteLine($"Area: {shape.CalculateArea()}");
Console.WriteLine($"Perimeter: {shape.CalculatePerimeter()}");
}
public static void Main()
{
// LSP implementation: shape is of circle type
Shape circle = new Circle(5.0);
// LSP implementation: shape is of rectangle type
Shape rectangle = new Rectangle(4.0, 6.0);
Console.WriteLine("Circle Details:");
PrintShapeDetails(circle);
Console.WriteLine("\nRectangle Details:");
PrintShapeDetails(rectangle);
}
}
Conclusion
The Liskov Substitution Principle is a pivotal concept in software design that fosters code extensibility, consistency, and polymorphism. By adhering to LSP, you create software that is more adaptable and less prone to bugs when new derived classes are introduced. While it requires careful consideration and rigorous testing, the long-term benefits of maintaining LSP in your codebase are well worth the effort. Embrace LSP, and elevate your software design practices to a higher level of excellence.
Комментарии