Title: Mastering Software Design with the Interface Segregation Principle (ISP)
Introduction
In the realm of software design, creating flexible and maintainable code is of paramount importance. One of the fundamental principles that can help achieve this goal is the Interface Segregation Principle (ISP). Originally introduced as part of the SOLID design principles, the ISP focuses on the importance of clean and efficient interfaces. In this article, we will delve into the Interface Segregation Principle, understand its significance, and explore how it can transform your software design practices.
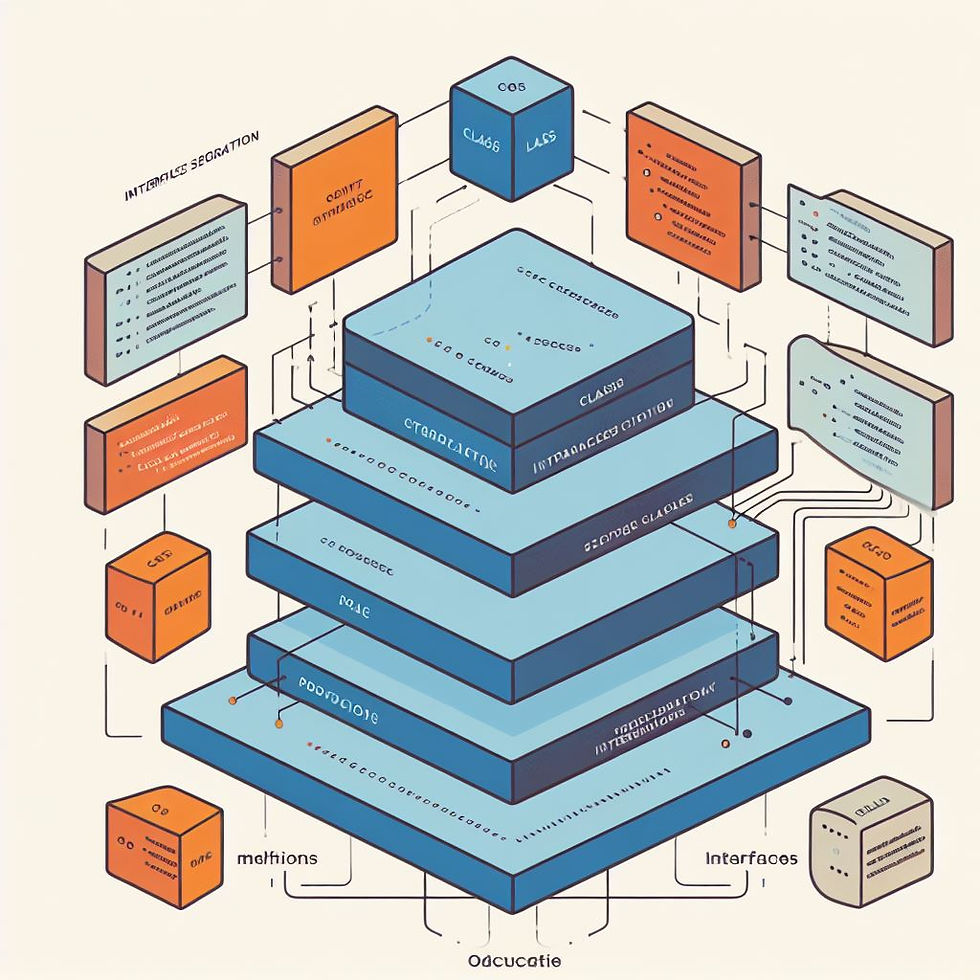
What is the Interface Segregation Principle (ISP)?
The Interface Segregation Principle is one of the five SOLID principles of object-oriented design. It can be summarized as follows: Clients should not be forced to depend on interfaces they do not use. In simpler terms, it emphasizes that interfaces should be tailored to specific use cases and not contain unnecessary methods that clients won't need.
Key Benefits of ISP
Enhanced Code Flexibility: Adhering to the ISP allows you to create more focused and specialized interfaces. This leads to greater code flexibility, as clients can implement only the methods they require, avoiding the burden of unused functionalities.
Improved Maintainability: Specialized interfaces make the codebase more maintainable. When changes or updates are needed, you can modify individual interfaces without affecting unrelated parts of the code.
Reducing Code Bloat: By avoiding large, monolithic interfaces, the ISP helps in reducing code bloat and complexity. This, in turn, simplifies code comprehension and development.
Encouraging Reusability: Smaller, specialized interfaces encourage code reusability. Clients can easily implement interfaces in various contexts without being weighed down by unnecessary methods.
How to Apply ISP in Your Code
Identify Specific Use Cases: Begin by identifying the distinct use cases for each interface. Consider what methods are genuinely required for each client.
Create Specialized Interfaces: Design interfaces that cater to specific use cases, keeping them as small and focused as possible. Avoid including methods that are not essential for a particular client.
Implement Multiple Interfaces: Clients should implement multiple specialized interfaces to fulfill their needs. This allows for fine-grained control and flexibility.
Refactor Existing Code: When applying the ISP to an existing codebase, be prepared to refactor and reorganize interfaces. Break down large interfaces into smaller, more focused ones to align with the principle.
Real-World Example
Let's consider a real-world example in a modern e-commerce system. Instead of having a single, all-encompassing "Payment" interface that includes methods for processing payments, refunding, and tracking orders, you can create distinct interfaces like "PaymentProcessor" and "OrderTracker." This segregation allows different components of the system, such as the checkout process and order management, to implement only the interfaces they need, improving code efficiency and maintainability.
using System;
public interface IPayment
{
void ProcessPayment(decimal amount);
void RefundPayment(decimal amount);
void TrackOrder(int orderId);
}
public interface IPaymentProcessor
{
void ProcessPayment(decimal amount);
void RefundPayment(decimal amount);
}
public interface IOrderTracker
{
void TrackOrder(int orderId);
}
public class CreditCardPayment : IPayment
{
public void ProcessPayment(decimal amount)
{
// Implement credit card payment processing logic
}
public void RefundPayment(decimal amount)
{
// Implement credit card refund logic
}
public void TrackOrder(int orderId)
{
// Implement order tracking logic for credit card payments
}
}
public class PayPalPayment : IPayment
{
public void ProcessPayment(decimal amount)
{
// Implement PayPal payment processing logic
}
public void RefundPayment(decimal amount)
{
// Implement PayPal refund logic
}
public void TrackOrder(int orderId)
{
// Implement order tracking logic for PayPal payments
}
}
Conclusion
The Interface Segregation Principle is a crucial concept in software design that promotes code flexibility, maintainability, and reusability. By adhering to the ISP, you can create software interfaces that are tailored to specific client needs, reducing code bloat and complexity. Although it may require some refactoring and restructuring, the long-term benefits are well worth the effort. Embrace the ISP, and elevate your software design practices to a new level of excellence.
Comments