Title: Unlocking Software Design Excellence with the Open-Closed Principle (OCP)
Introduction
In the dynamic world of software development, creating flexible and extensible code is essential. The Open-Closed Principle (OCP) is one of the SOLID principles that can significantly contribute to this goal. In this article, we will delve into the Open-Closed Principle, understand its significance, and explore how it can elevate your code to new heights.
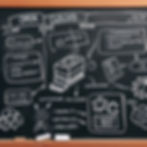
What is the Open-Closed Principle (OCP)?
The Open-Closed Principle is one of the five SOLID principles of object-oriented design, introduced by Bertrand Meyer. In essence, it states that software entities (such as classes, modules, or functions) should be open for extension but closed for modification. This principle encourages developers to design their software in a way that allows for the addition of new features or functionalities without altering existing, working code.
Key Benefits of OCP
Code Stability: By following the Open-Closed Principle, you can make changes to your codebase without introducing new bugs or causing unintended side effects in existing, tested functionality. This leads to greater code stability and fewer disruptions in your software.
Reusability: OCP promotes the creation of flexible, extensible components that can be reused across different parts of your application or in entirely new projects. This reusability reduces redundancy, saves development time, and ensures consistency.
Scalability: As your software grows and evolves, OCP allows you to add new features or capabilities without rewriting existing code. This adaptability is crucial for building scalable and future-proof systems.
Reduced Technical Debt: The Open-Closed Principle helps to keep technical debt in check. By adhering to OCP, you minimize the need for constant code refactoring, which can become a source of technical debt if not managed properly.
How to Apply OCP in Your Code
Abstraction and Interfaces: Utilize abstraction and interfaces to create a stable contract for your software entities. This allows extensions to be implemented through new classes that adhere to the same interface, without modifying existing code.
Dependency Injection: Implement the principle of dependency injection to pass dependencies into your classes rather than hard-coding them. This makes it easier to replace or extend functionality without altering the existing codebase.
Use Design Patterns: Explore design patterns such as the Strategy Pattern, Decorator Pattern, or Factory Pattern, which can help you implement OCP in your software architecture. These patterns facilitate the addition of new functionality without modifying existing classes.
Test-Driven Development (TDD): Consider adopting Test-Driven Development practices. Writing tests before writing code helps ensure that your existing functionality remains unchanged when extending your software.
Real-World Example
Imagine you are developing an e-commerce application, and you need to support various payment methods. Instead of modifying the existing PaymentProcessor class whenever a new payment method is introduced, you can apply the Open-Closed Principle. Create new payment method classes that adhere to a common payment interface and implement their specific functionality. Your codebase remains closed for modification but open for extension.
// Base interface for payment methods
public interface IPaymentMethod
{
void ProcessPayment(decimal amount);
}
// Existing PaymentProcessor class
public class PaymentProcessor
{
public void ProcessPayment(IPaymentMethod paymentMethod, decimal amount)
{
paymentMethod.ProcessPayment(amount);
}
}
// New payment methods
public class CreditCardPayment : IPaymentMethod
{
public void ProcessPayment(decimal amount)
{
// Implement credit card payment processing logic
}
}
public class PayPalPayment : IPaymentMethod
{
public void ProcessPayment(decimal amount)
{
// Implement PayPal payment processing logic
}
}
public class BitcoinPayment : IPaymentMethod
{
public void ProcessPayment(decimal amount)
{
// Implement Bitcoin payment processing logic
}
}
Conclusion
The Open-Closed Principle is a cornerstone of solid software design. By adhering to OCP, you can create resilient, extensible, and adaptable code to changing requirements. It allows you to evolve your software without constantly modifying existing code, resulting in more efficient development and a reduced risk of introducing bugs. Embrace OCP, and you'll be well on your way to achieving software design excellence.