Understanding Onion Architecture
- amol ankit
- Jun 18, 2024
- 4 min read
Understanding Onion Architecture: A Guide for New Software Developers
Starting a career as a software developer opens up opportunities to delve into various architectural patterns that can significantly impact the quality and maintainability of your applications. Among the plethora of architectural patterns, the Onion Architecture stands out as a structured approach that can guide your development towards building robust and scalable software solutions.
Onion Architecture is a design pattern that emphasizes separating concerns within a software application. This architectural style promotes modularity, testability, and flexibility in projects by structuring the codebase into distinct layers, with dependencies flowing inwards towards the core.
Understanding the core principles of Onion Architecture is essential for any aspiring software developer. The clear distinction between the innermost core, which contains business logic and domain models, and the outer layers responsible for infrastructure and user interface interactions ensures a clean separation of responsibilities.
Implementing Onion Architecture in your projects involves carefully considering how different components interact and depend on each other. By following the dependency inversion principle and decoupling high-level policies from low-level details, you can achieve a design that is easy to maintain and allows for seamless integration of new features and technologies.
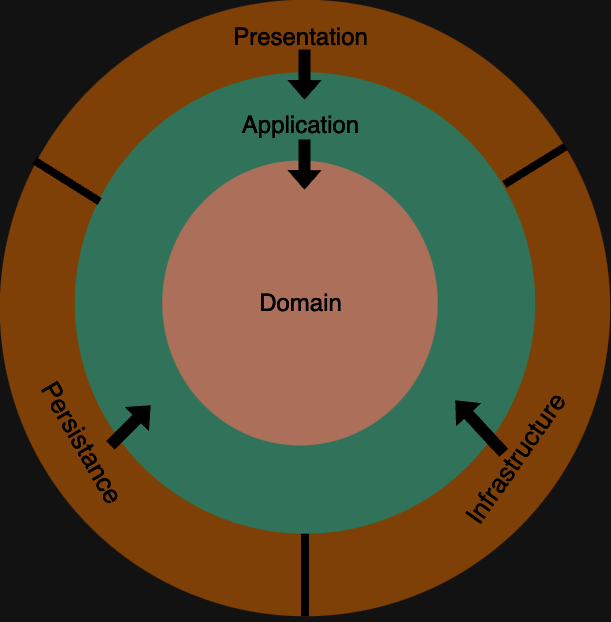
What is Onion Architecture?
Onion Architecture, introduced by Jeffrey Palermo in 2008, is a software architecture pattern designed to address common issues in software development, such as tight coupling and separation of concerns. By organizing code into layers with clear dependencies, this architecture creates a system that is easy to maintain, test, and extend.
Key Principles of Onion Architecture
Dependency Inversion: High-level modules should not depend on low-level modules. Both should depend on abstractions.
Separation of Concerns: Different aspects of the application should be separated into distinct layers.
Center-to-Outer Dependency Rule: The inner layers should not depend on the outer layers; instead, the outer layers should depend on the inner layers.
Layers of Onion Architecture
Onion Architecture is typically visualized as a series of concentric circles (like an onion), with each layer representing a different level of abstraction. Here’s a breakdown of the layers from the core to the outermost:
Domain Layer (Core)
The domain layer is the heart of the Onion Architecture. It contains the business logic and domain entities, which represent the application's core concepts.
Entities: These are the fundamental building blocks of the business model, usually represented by classes.
Value Objects: Immutable objects that represent descriptive aspects of the domain with no identity.
Domain Services: Operations that don't naturally fit within a single entity and typically span multiple entities.
Application Layer
The application layer contains the application-specific business rules. It coordinates the activities of the domain layer but does not contain any business logic itself.
Use Cases: These are specific operations or actions the application can perform, implementing the application's business logic by interacting with domain entities.
Infrastructure Layer
The infrastructure layer contains technical details and implementations such as data access, external services, and other low-level concerns.
Repositories: Abstractions over data storage, providing methods to retrieve and persist domain entities.
External Services: Interfaces and implementations for external APIs and services.
Presentation Layer (User Interface)
The presentation layer is responsible for presenting the information to the user and interpreting user commands. This layer interacts with the application layer to perform actions and display results.
Controllers: Handle user inputs and translate them into actions on the application layer.
Views: Render data to the user and handle user interactions.
Benefits of Onion Architecture
Testability: By isolating the core business logic from external concerns, you can easily write unit tests for the domain and application layers.
Maintainability: Clear separation of concerns makes managing and modifying the codebase easier.
Flexibility: The architecture allows you to change external layers (e.g., database, UI) without affecting the core business logic.
Implementing Onion Architecture: A Step-by-Step Guide
Let's describe a simple example of implementing Onion Architecture in a .NET application.
Define the Domain Layer
public class Customer
{
public int Id { get; private set; }
public string Name { get; private set; }
public Customer(int id, string name)
{
Id = id;
Name = name;
}
// Business logic methods
}
public interface ICustomerRepository
{
Customer GetById(int id);
void Save(Customer customer);
}
Define the Application Layer
public class CustomerService
{
private readonly ICustomerRepository _customerRepository;
public CustomerService(ICustomerRepository customerRepository)
{
_customerRepository = customerRepository;
}
public void UpdateCustomerName(int customerId, string newName)
{
var customer = _customerRepository.GetById(customerId);
if (customer == null)
throw new Exception("Customer not found");
customer.UpdateName(newName); // Business logic method
_customerRepository.Save(customer);
}
}
Implement the Infrastructure Layer
public class CustomerRepository : ICustomerRepository
{
// Assume we have a DbContext (Entity Framework) injected
public Customer GetById(int id)
{
// Retrieve customer from database
}
public void Save(Customer customer)
{
// Save customer to database
}
}
Implement the Presentation Layer
[ApiController]
[Route("api/[controller]")]
public class CustomerController : ControllerBase
{
private readonly CustomerService _customerService;
public CustomerController(CustomerService customerService)
{
_customerService = customerService;
}
[HttpPut("{id}")]
public IActionResult UpdateCustomerName(int id, [FromBody] string newName)
{
_customerService.UpdateCustomerName(id, newName);
return Ok();
}
}
Conclusion
Onion Architecture provides a robust framework for developing maintainable, testable, and flexible applications by promoting the separation of concerns and adhering to the dependency inversion principle. As a new developer, understanding and implementing this architecture can significantly improve the quality and longevity of your software projects. Organizing your code into clearly defined layers ensures your application remains adaptable to changing requirements and technologies.
Commentaires