Test-driven Development (TDD) is a software development approach where tests are written before the actual code. The development process in TDD typically follows a cycle known as the "Red-Green-Refactor" cycle. Here's an explanation of each step in the context of C#:
Red: In this phase, you write a failing test. This test represents the desired behaviour of the code you're about to write.
Green: In this phase, you write the minimum code necessary to pass the failing test. The goal is to implement the functionality required by the test.
Refactor: Once the test passes, you can refactor the code to improve its structure, readability, or performance while ensuring the tests still pass.
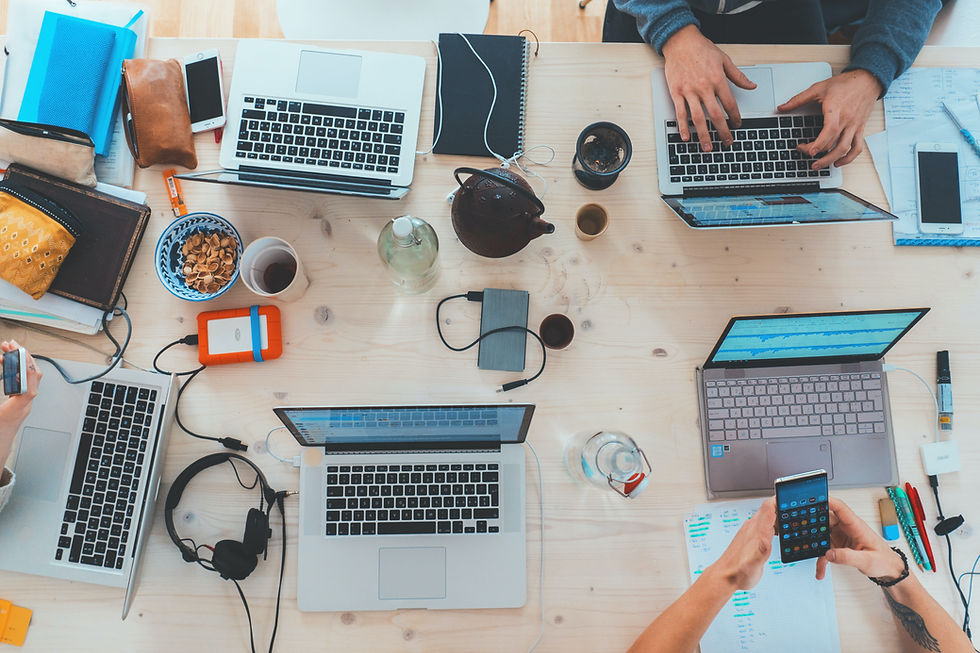
Let's go through an example in C# step by step:
Step 1: Create a Test (Red)
[TestClass]
public class CalculatorTests
{
[TestMethod]
public void Add_TwoNumbers_ReturnsSum()
{
// Arrange
Calculator calculator = new Calculator();
// Act
int result = calculator.Add(2, 3);
// Assert
Assert.AreEqual(5, result); // This test will fail initially
}
}
Step 2: Make the Test Pass (Green)
public class Calculator
{
public int Add(int a, int b)
{
return a + b;
}
}
[TestClass]
public class CalculatorTests
{
[TestMethod]
public void Add_TwoNumbers_ReturnsSum()
{
// Arrange
Calculator calculator = new Calculator();
// Act
int result = calculator.Add(2, 3);
// Assert
Assert.AreEqual(5, result); // This test will pass now
}
}
Step 3: Refactor (Optional)
// No refactoring is needed in this simple example, but you might refactor for more complex code
Benefits of Test-Driven Development
Test-Driven Development (TDD) offers several benefits that contribute to software development's overall quality, maintainability, and efficiency. Here are some key advantages of practising TDD:
Early Bug Detection:- TDD helps catch bugs and defects early in Development. By writing tests before the actual code, developers must consider the expected behaviour and potential issues upfront.
Improved Code Quality:- TDD encourages writing modular, clean, and maintainable code. Developers focus on creating code that passes the tests and follows best practices, resulting in higher code quality.
Maintainability and Refactoring:- With a comprehensive suite of tests, developers can refactor their code confidently. If any issues are introduced during Refactoring, the tests will catch them, ensuring the code remains functional.
Regression Testing:- TDD provides a suite of automated tests that can be easily executed whenever changes are made to the codebase. This helps prevent regressions, ensuring new features or modifications don't break existing functionality.
Design Improvement:- TDD encourages a more modular and loosely coupled design. Writing tests first forces developers to consider the interfaces and interactions between different components, leading to more flexible and scalable architectures.
Documentation:- Tests serve as a form of documentation for the codebase. They provide a clear specification of the expected behaviour of each component, making it easier for other developers (or even the original developer) to understand and work with the code.
Increased Developer Confidence:- TDD provides developers with a safety net. Knowing that changes are unlikely to introduce defects that go unnoticed, developers gain confidence in their code, making them more willing to implement new features or improve.
Faster Development in the Long Run: TDD may seem to slow down the initial development process, but it often leads to speedier Development in the long run. The time saved on debugging and fixing issues outweighs the time spent writing tests upfront.
Collaboration:- TDD promotes collaboration between team members. Tests provide a clear specification of requirements, making it easier for different team members to work on different parts of the system simultaneously.
Customer Satisfaction:- TDD contributes to a more stable and reliable product. By reducing the number of defects and ensuring that changes don't break existing functionality, TDD helps deliver a more consistent and satisfying user experience.
Best Practice for Writing Tests
Here are some key practices to consider when practising TDD
Write the Simplest Code to Pass the Test:- Only write as much code as needed to make the failing test pass. Please resist the temptation to add unnecessary complexity or features until required.
Write a Single Test Case at a Time:- Focus on one test case at a time. This helps maintain a clear understanding of the expected behaviour and simplifies debugging if a test fails.
Run Tests Frequently:- Run your tests frequently, ideally after every small Change. This ensures that issues are identified quickly, and you can be confident that your code is functioning as expected.
Refactor Safely:- Refactor the code to improve its design or readability after a test passes. The tests act as a safety net, ensuring that any changes don't introduce defects.
Keep Tests and Code in Sync:- Ensure that tests are updated whenever code changes are made, and vice versa. This prevents the codebase from becoming outdated and inaccurate.
Use Descriptive Test and Method Names:- Write clear and descriptive names for your tests and methods. This makes it easier for other developers to understand the purpose of each test and method.
Isolate Tests:- Tests should be independent of each other. Avoid dependencies between tests, as this can lead to false positives or negatives when running tests.
Test Edge Cases: Cover common scenarios and edge cases in your tests. This helps ensure that your code behaves correctly in various situations.
Keep Tests Fast:- Tests should run quickly to encourage frequent execution. Slow tests can hinder the TDD process and reduce developers' likelihood of running them regularly.
Use Test Doubles:- Employ test doubles (mocks, stubs, etc.) to isolate the code under test. This allows you to focus on testing the specific behaviour of the unit without relying on external dependencies.
Continuous Integration (CI):- Integrate TDD into your continuous integration process. Set up automated builds and tests to run whenever code is pushed to the repository, ensuring the entire codebase remains functional.
Emphasize the Red-Green-Refactor Cycle:- Follow the Red-Green-Refactor cycle strictly. Write a failing test (Red), make the test pass (Green), and then refactor the code while keeping the tests passing (Refactor).
Communicate Intent Through Tests:- Tests serve as documentation for the code. Ensure that your tests communicate the intent and expected behaviour of your code.
How does TDD fit in Agile?
TDD aligns well with the principles of Agile by promoting iterative Development, collaboration, and a focus on delivering high-quality, maintainable software. Here's how TDD fits into Agile development
Iterative Development:- Agile Development emphasizes iterative and incremental Development. TDD is inherently iterative, as it follows the Red-Green-Refactor cycle. Developers write small increments of code, accompanied by corresponding tests, and continuously refine the codebase.
Early and Continuous Feedback:- TDD provides instant feedback on the correctness of the code. By writing tests before the actual code, developers receive immediate feedback on whether their changes meet the expected requirements. This aligns with Agile's principle of obtaining early and continuous feedback.
Adaptability to Change:- Both TDD and Agile value the ability to adapt to changing requirements. TDD makes it easier to accommodate changes by providing a safety net for tests. When conditions change, developers can confidently refactor the code, knowing the tests will catch any regressions.
Collaboration and Communication:- TDD encourages collaboration between developers, testers, and other stakeholders. Tests serve as executable specifications everyone can understand, fostering effective Communication within Agile cross-functional teams.
Quality Focus:- Agile Development emphasizes delivering a high-quality product. TDD contributes to this goal by ensuring that code is thoroughly tested and that regressions are minimized. The emphasis on writing tests first helps create more robust and maintainable code.
Continuous Integration (CI) and Continuous Deployment (CD):- TDD integrates seamlessly with CI/CD practices commonly found in Agile environments. Automated tests created through TDD are ideal for inclusion in continuous integration pipelines, where they can be run automatically whenever new code is added.
Reduced Technical Debt:- Agile aims to minimize technical debt, and TDD is a valuable tool for achieving this. Developers can maintain a clean codebase by continually refactoring and ensuring that tests cover new code and avoid accumulating technical debt.
Customer Satisfaction:- Agile is customer-centric, and TDD contributes to customer satisfaction by reducing the number of defects and ensuring that the software meets the specified requirements. Reliable tests allow for more confident delivery of features, reducing the likelihood of unexpected issues.
Short Feedback Loops:- Both TDD and Agile emphasize short feedback loops. TDD provides quick feedback on the correctness of the code, allowing developers to identify and address issues early in the development process.
Conclusion
The idea is to repeat this cycle for each new piece of functionality. By following TDD, you ensure that your code is thoroughly tested and any changes made to the code won't introduce unexpected issues. The tests act as a safety net, allowing you to make modifications confidently.
Comentarios