Mastering Software Design with the Single Responsibility Principle (SRP)
Introduction
In the world of software development, creating clean, maintainable, and scalable code is paramount. One of the fundamental principles that can help you achieve this is the Single Responsibility Principle (SRP). This principle is a key component of the SOLID design principles, originally introduced by Robert C. Martin. In this article, we'll dive deep into the Single Responsibility Principle, understand its importance, and explore how it can transform your codebase for the better.
What is the Single Responsibility Principle (SRP)?
The Single Responsibility Principle is one of the five SOLID principles of object-oriented design. It can be summarized as follows: A class should have only one reason to change. In other words, a class should have only one primary responsibility. This principle encourages the separation of concerns, making your code more modular and easier to maintain.
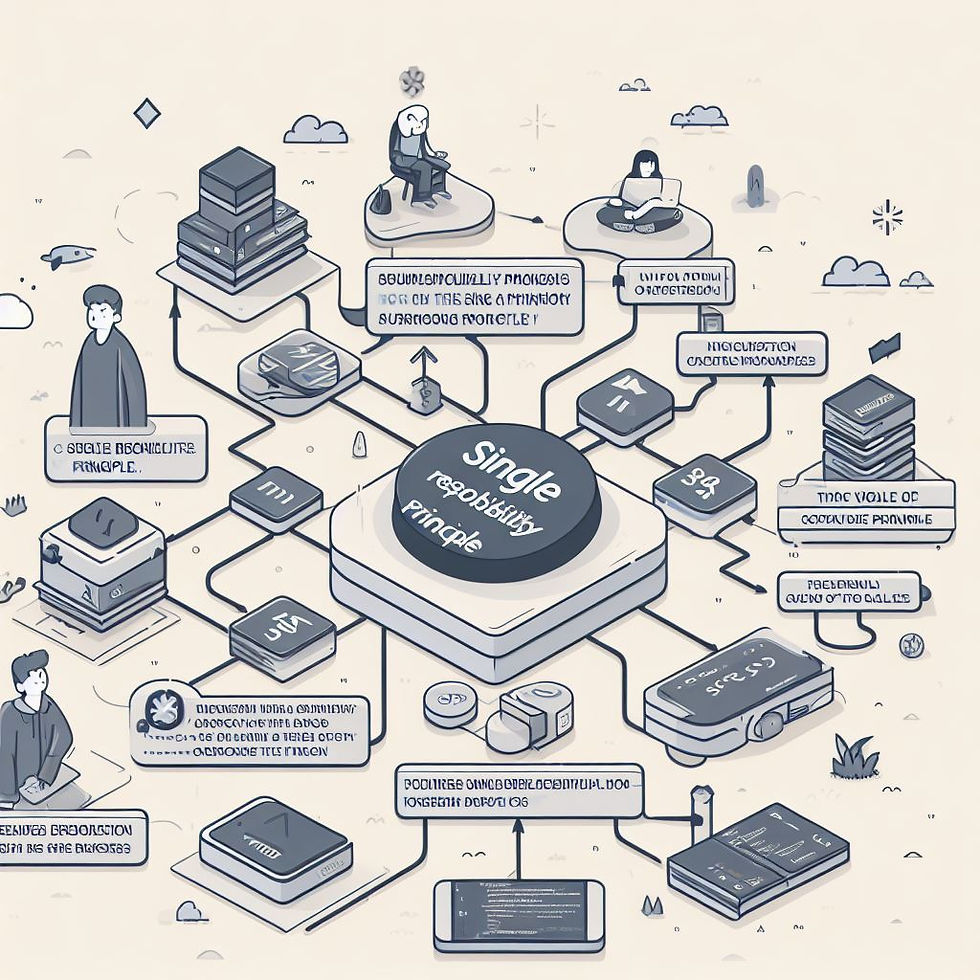
Key Benefits of SRP
Improved Code Maintainability: When you follow the SRP, each class has a single, well-defined purpose. This makes it easier to locate and fix issues, add new features, and understand the codebase, leading to improved code maintainability.
Enhanced Code Reusability: A class with a single responsibility is more likely to be reusable in different parts of your application or entirely new projects. This reduces the need for duplicating code, which can lead to a reduction in bugs and increased development efficiency.
Easier Testing: SRP promotes smaller, focused classes, which are easier to test. You can write unit tests for individual classes without the need for complex setup or testing multiple concerns at once. This results in more robust testing and fewer test cases.
Scalability: As your application grows, adhering to SRP allows you to scale your codebase more effectively. You can add new functionality or make changes without affecting the existing code, minimizing the risk of introducing bugs in unrelated areas.
How to Apply SRP in Your Code
Identify Responsibilities: Start by identifying the distinct responsibilities within your codebase. This could be as simple as extracting a group of methods into a new class or creating a separate module to handle a specific task.
Create Smaller Classes: Once you've identified responsibilities, create smaller classes, each responsible for a single task. Avoid creating "God objects" that try to do everything.
Maintain a Clear Interface: Ensure that the interface of each class or module clearly defines its purpose. Naming conventions should be descriptive and indicative of what each component does.
Minimize Dependencies: Reduce dependencies between classes to maintain the separation of concerns. This will make changing one part of your code easier without affecting other parts.
Real World Example
Let's consider the below example of a Book class
public class Book
{
privatestring _bookName;
privatestring _authorName;
privatestring _text;
//
Remaining Implementation
......
//
public string ReplaceText(string word, string replaceWith)
{
return_text.Replace(word,replaceWith);
}
public bool WordPresentInBook(string word)
{
return_text.contains(word);
}
publicvoidPrintText()
{
Console.WriteLine(_text);
}
}
Now at first glance, the class above seems fine and there are no issues with it.
But consider this scenario, what if in future we want to add new features to the Printing of the text?
In the context of the Book class, it should only deal with stuff related to the core functionality of a book.
So, how can we fix this?
It's simple just remove the logic of printing the text to a separate class which only deals with Books IO operation and keep the core implementation in the Book class.
public class PrintBook
{
// Constructor and rest of the implemntation
public void PrintTextToConsole()
{
// Implementation
}
public void OtherPrinitngMethods()
{
// Implementation
}
}
Conclusion
The Single Responsibility Principle is a crucial concept in software design that can significantly improve the quality of your code. By adhering to SRP, you'll create code that is easier to maintain, more reusable, and better prepared for future expansion. Implementing SRP may require some refactoring and restructuring, but the long-term benefits are well worth the effort. So, embrace SRP, and watch your software design skills and codebase flourish.
Comments