Unity with the Composite Pattern
Greetings, fellow developers! Today, we embark on a journey through the lush landscapes of design patterns, with our focus set on the versatile and powerful Composite Pattern with C#. If you've ever found yourself yearning for a way to seamlessly handle individual objects and their compositions within a unified structure, the Composite Pattern is the beacon guiding you through the forest of software design.
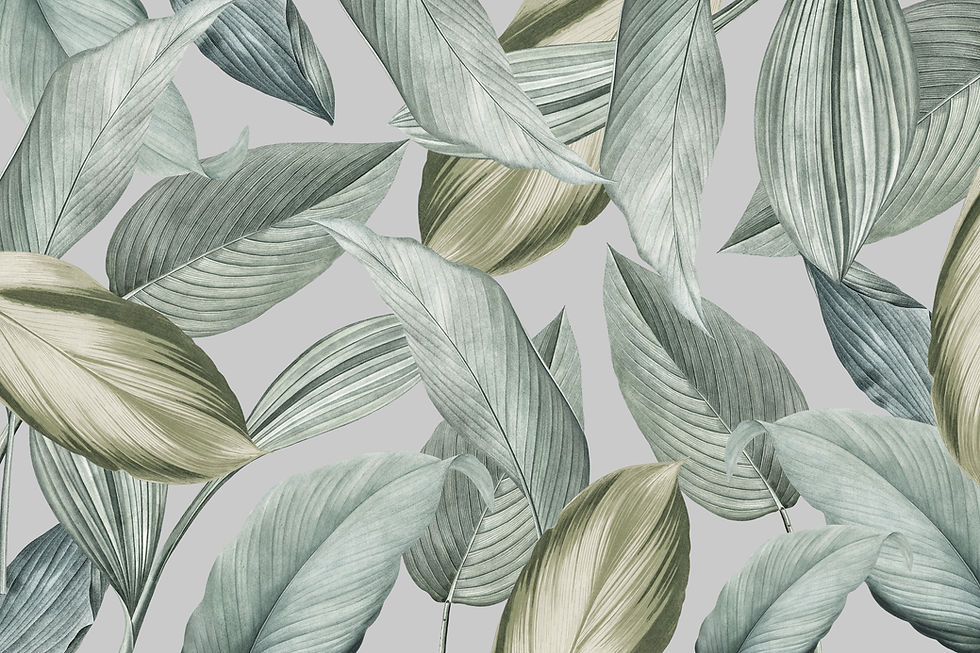
The Art of Unity: Components, Leafs, and Composites
Component Interface: IGraphic
Let's start our journey by defining the common ground, the `IGraphic` interface:
public interface IGraphic
{
void Draw();
}
This interface serves as the foundation for both individual elements and their compositions, ensuring a consistent way to invoke the `Draw` method.
Leaf: Circle
Next, we encounter the individual elements represented by the `Circle` class:
public class Circle : IGraphic
{
public void Draw()
{
Console.WriteLine("Drawing Circle");
}
}
The `Circle` class, implementing the `IGraphic` interface, stands as a leaf in our design tree - a singular, drawable entity.
Composite: CompositeGraphic
Our journey reaches a critical point with the `CompositeGraphic` class:
public class CompositeGraphic : IGraphic
{
private List<IGraphic> graphics = new List<IGraphic>();
public void Add(IGraphic graphic)
{
graphics.Add(graphic);
}
public void Remove(IGraphic graphic)
{
graphics.Remove(graphic);
}
public void Draw()
{
Console.WriteLine("Drawing Composite Graphic");
foreach (var graphic in graphics)
{
graphic.Draw();
}
}
}
`CompositeGraphic` is our composite, capable of holding both individual `Circle` objects and other `CompositeGraphic` instances. Through the `Add`, `Remove`, and `Draw` methods, it orchestrates a symphony of drawing actions within its unified structure.
Embracing Unity in Code
Let's put the Composite Pattern to the test:
var composite = new CompositeGraphic();
composite.Add(new Circle());
composite.Add(new Circle());
composite.Add(new Circle());
// Drawing the composite, which in turn draws all its children
composite.Draw();
Here, we create a `CompositeGraphic`, populate it with individual `Circle` elements, and witness the magic as the `Draw` method is called. The Composite Pattern seamlessly unifies the drawing process for both individual elements and their compositions.
Conclusion: Crafting Harmony in Code
As we wrap up our exploration of the Composite Pattern in C#, we celebrate its ability to create unity within diversity. Whether you're orchestrating graphical elements or navigating the intricacies of software design, the Composite Pattern stands as a testament to the art of unification.
Nice