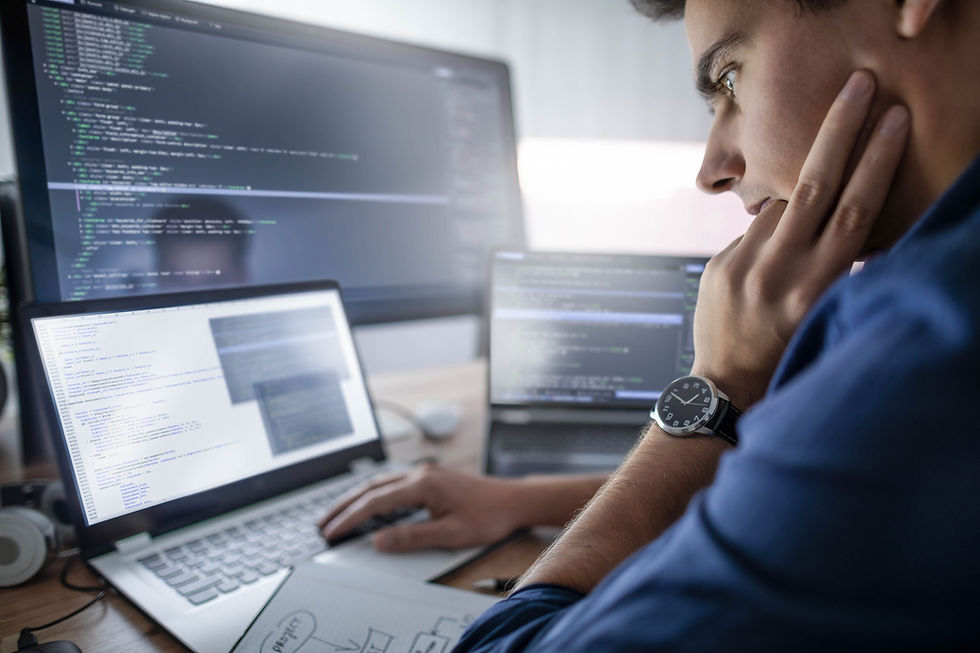
SOLID principles are a collection of 5 different practices which should be followed if you are to create a robust and reliable software code base/classes.
These five principles serve as a foundation for comprehending the significance of specific design patterns and software architecture as a whole. Consequently, I firmly hold the belief that they constitute essential knowledge for every developer.
Below I will be trying to explain each of the principles one by one so that you can understand and will feel a bit more confident while implementing them in your project.
Background
SOLID principles were introduced by Robert C. Martin, often referred to as "Uncle Bob."
Uncle Bob is also the author of the bestselling books "Clean Code" and "Clean Architecture".
NB: There is no specific order to the principles it can be DOLIS or DILOS.
Details of each SOLID principle
Single Responsibility Principle
Single Responsibility Principle: This principle states that a class should only have one reason for change and
should only be doing one thing and one thing alone.
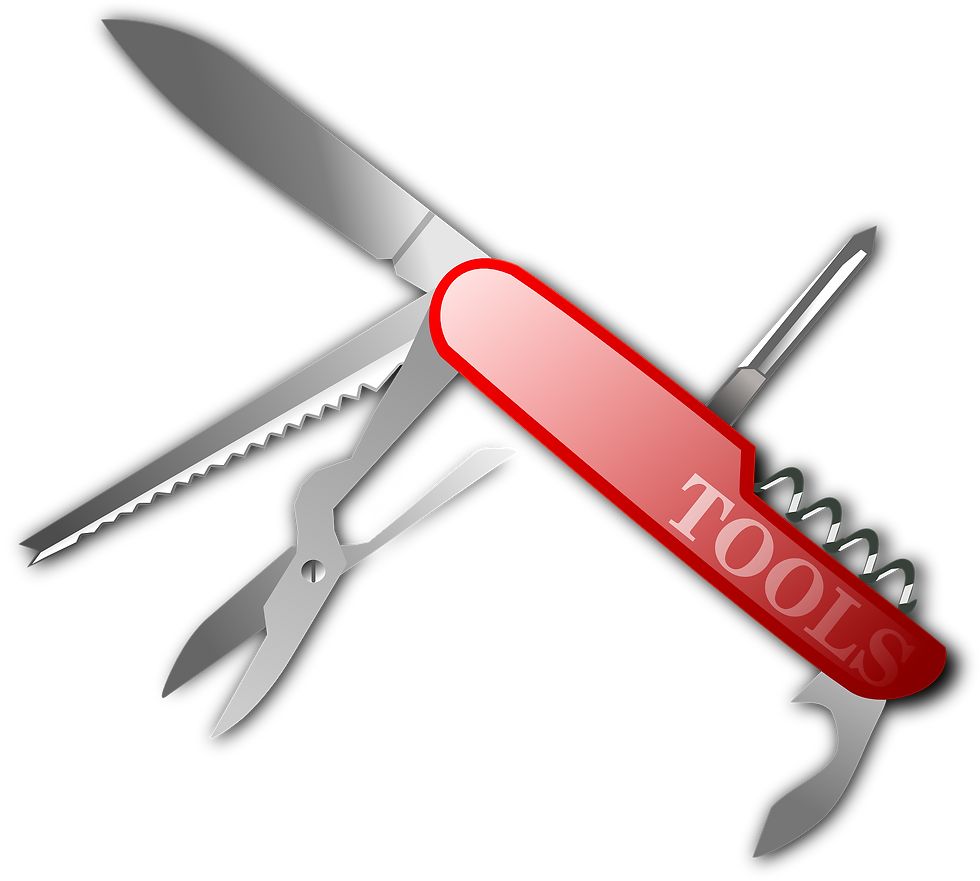
For those who don't know above image is of a Swiss knife a multipurpose tool, no doubt that this is a very useful tool if you are camping or doing some similar activity.
However, the software development world is a bit different from our normal world. In the software development world the simpler the better, and you should have specific tools for specific purposes.
How does it work?
Let's see with example.
public class Book
{
private string _bookName;
private string _authorName;
private string _text;
// Remaining Implementation
...
...
//
public string ReplaceText(string word, string replacementWord)
{
return _text.Replace(word, replacementWord);
}
public boolean WordPresentInBook(string word)
{
return _text.contains(word);
}
public void PrintText()
{
Console.WriteLine(_text);
}
}
Can you guess what is the issue here?
Now at first glance, the class above seems fine and there are no issues with it.
But If you guessed the PrintText method is the violation then you are absolutely correct and you get the crux of this principle.
For those who didn't, Let's explore it in detail.
Consider this scenario, what if in future you want to print the text of the book via. method call to a printer or want to print the text with some other medium?
In the context of the Book class, it should only deal with stuff related to the core functionality of a book.
So, how can we fix this?
It's simple just remove the logic of printing the text to a separate class which only deals with Books IO operation and keep the core implementation in the Book class.
public class PrintBook
{
// Constructor and rest of the implemntation
public void PrintTextToConsole()
{
// Implementation
}
}
Open Closed Principle
Liskov substitution Principle
Interface Segregation Principle
Dependency Inversion Principle
Conclusion
In conclusion, the SOLID principles are not just a set of abstract guidelines; they are fundamental pillars that underpin good software design. By adhering to these principles, developers can create code that is clean, maintainable, and adaptable. In the ever-evolving world of software development, SOLID provides a reliable framework for building robust and efficient systems, ultimately leading to higher-quality software, improved collaboration among development teams, and greater customer satisfaction.
As you apply these principles in your software development journey, remember that they are not rigid rules but rather flexible guidelines to help you make informed design decisions. The SOLID principles empower you to write code that can stand the test of time and evolve with changing requirements. They are an invaluable asset for any developer looking to elevate their skills and craft software that not only works but works well.
Comments