Adapt your code with the Adapter Design Pattern
- amol ankit
- Nov 19, 2023
- 3 min read
Unveiling the Power of the Adapter Design Pattern in Software Development
Introduction:
The world of software design is filled with challenges, and as developers, our goal is to create robust, flexible, and maintainable systems. To achieve this, we often turn to design patterns – proven solutions to common problems. One such design pattern that comes to the rescue in scenarios where systems need to work together seamlessly is the Adapter Design Pattern.
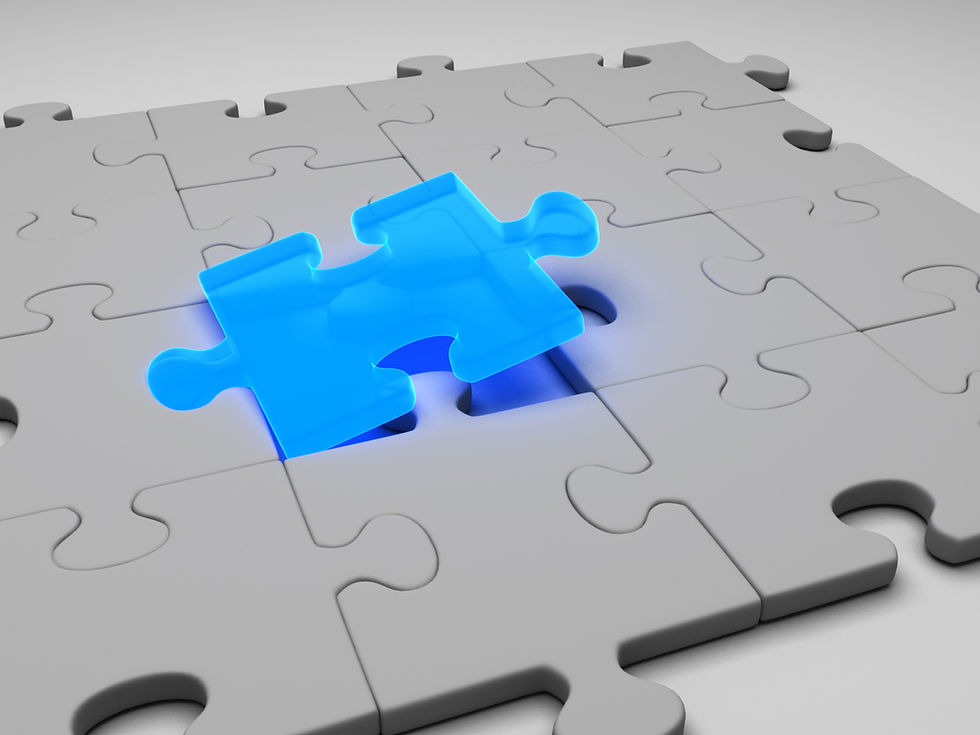
Understanding the Adapter Design Pattern:
What is the Adapter Design Pattern?
The Adapter Design Pattern is a structural pattern that allows incompatible interfaces to work together. It acts as a bridge between two incompatible interfaces by converting the interface of a class into another interface that a client expects. In essence, it makes two incompatible interfaces compatible.
When to Use the Adapter Pattern?
Integration of Legacy Systems: When integrating new systems with existing legacy code, you may encounter incompatible interfaces. The Adapter Pattern can help make these systems work together without modifying their source code.
Reusability: Adapters can be handy when you want to reuse a class but its interface is not compatible with the rest of your application. Instead of modifying the existing code, you can create an adapter to make it compatible.
Third-Party Libraries: When using third-party libraries with different interfaces, the Adapter Pattern can be employed to create a uniform interface, making it easier to work with various libraries in the same project.
Components of the Adapter Design Pattern:
Target: This defines the interface that the client code expects. It's the interface the client interacts with.
Adaptee: This is the existing interface that needs to be adapted. It's the interface that the client can't use directly.
Adapter: The Adapter is the bridge between the Target and the Adaptee. It implements the Target interface and holds an instance of the Adaptee. It translates the client requests into a format that the Adaptee can understand.
Steps to implement the Adapter design pattern
Identify two classes with incompatible interface implementation.
Declare the client class and define how it will communicate with the service.
Create the Adapter and make it follow the client interface leaving all the methods empty.
Initialize a field in the adapter class concerning the service object. The service object is an adaptee. It can be a 3rd party class or a legacy class.
Implement the methods of the Client’s interface in the adapter class. Make sure that the Adapter only delegates the real work of the service object.
Make the Client use the Adapter class via the Client interface so that you can change the adapters without modifying the Client’s code.
Implementing the Adapter Design Pattern:
Let's consider a practical example of adapting a third-party weather service to our application. Suppose the third-party service provides weather information using an XML interface, but our application expects JSON data.
using System;
// Target interface
public interface IWeatherService
{
string GetWeatherData();
}
// Adaptee (third-party service with XML interface)
public class ThirdPartyWeatherService
{
public string FetchXMLData()
{
// Logic to fetch weather data in XML format
return "<weather><temperature>72°F</temperature></weather>";
}
}
// Adapter
public class WeatherServiceAdapter : IWeatherService
{
private readonly ThirdPartyWeatherService adaptee;
public WeatherServiceAdapter(ThirdPartyWeatherService adaptee)
{
this.adaptee = adaptee;
}
public string GetWeatherData()
{
// Translate XML data to JSON (simplified for the example)
string xmlData = adaptee.FetchXMLData();
// Logic to convert XML to JSON (simplified for the example)
string jsonData = ConvertXMLtoJSON(xmlData);
return jsonData;
}
private string ConvertXMLtoJSON(string xmlData)
{
// Logic to convert XML to JSON (simplified for the example)
// Implement your XML to JSON conversion logic here
return "{\"temperature\":\"22°C\"}";
}
}
// Client code
class Program
{
static void Main()
{
// Instantiate the ThirdPartyWeatherService
ThirdPartyWeatherService thirdPartyService = new ThirdPartyWeatherService();
// Create the adapter
IWeatherService weatherService = new WeatherServiceAdapter(thirdPartyService);
// Use the adapted service to get weather data
string jsonData = weatherService.GetWeatherData();
// Display the result
Console.WriteLine(jsonData);
}
}
In this example, the `WeatherServiceAdapter` acts as the adapter, allowing our application to seamlessly integrate with the third-party weather service.
Benefits of the Adapter Design Pattern:
Flexibility: The Adapter Pattern allows for flexibility in integrating new components into existing systems without altering their source code.
Reusability: Existing classes can be reused in new systems without extensive modifications.
Interoperability: The pattern promotes interoperability between different components, systems, or libraries with incompatible interfaces.
Conclusion:
The Adapter Design Pattern proves to be a valuable asset in scenarios where systems with incompatible interfaces need to collaborate. By providing a bridge between different interfaces, the Adapter Pattern fosters flexibility, reusability, and interoperability, ultimately contributing to the development of more maintainable and scalable software systems.
As developers, understanding and leveraging the power of design patterns like the Adapter Pattern empowers us to overcome challenges and build software that stands the test of time.
Interesting