Once upon a time in our digital kingdom, where algorithms danced in the moonlight and developers were free to roam, there was a powerful design pattern known as the Strategy Pattern. This pattern held the key to orchestrating a symphony of flexibility within software systems.
In the heart of this kingdom, a wise developer named John found himself faced with a formidable challenge. He was tasked with creating a system that could handle different payment methods seamlessly, adapting to the ever-changing needs of the kingdom's digital marketplace.
Enter the Strategy Pattern, a blueprint that would soon become his guide in weaving the intricate tapestry of his solution.
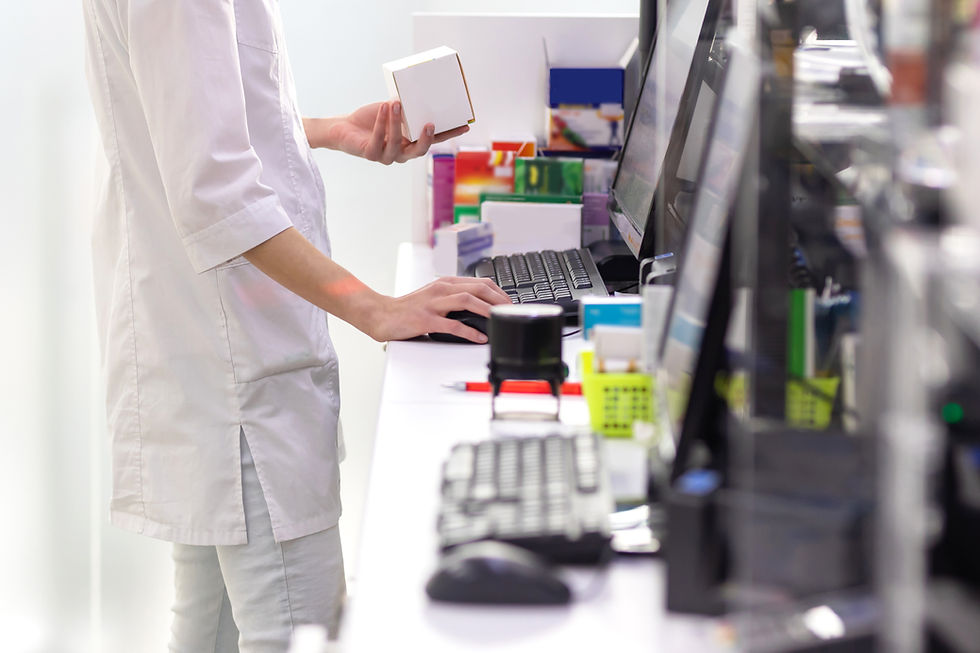
Act 1: The Ensemble of Strategies
In the vast realm of this kingdom, there existed three key characters: the Context, the Strategy Interface, and the Concrete Strategies.
The Context: The Context, a champion orchestrator named ShoppingCart, was entrusted with maintaining harmony in the payment process. This class held a mysterious reference to a Strategy Interface.
The Strategy Interface: The Strategy Interface, a beacon of guidance, declared the common methods that all Concrete Strategies must follow. It set the stage for the algorithms that would soon dance through the code.
The Concrete Strategies: Two enchanting dancers, CreditCardPayment and PayPalPayment, emerged as Concrete Strategies. They implemented the mesmerizing moves dictated by the Strategy Interface, each showcasing a unique algorithm for payment.
Act 2: The Dance of Flexibility
As the moon rose high in the digital sky, John wove the spell of flexibility using the Strategy Pattern. The ShoppingCart class, acting as the dance floor, gracefully accepted different payment partners, thanks to its Strategy Interface.
/// <summary>
/// The context class
/// </summary>
public class ShoppingCart
{
private IPaymentStrategy _paymentStrategy;
/// <summary>
/// Constructor
/// </summary>
/// <param name="paymentStrategy">Injected dependency payment strategy</param>
public ShoppingCart(IPaymentStrategy paymentStrategy)
{
_paymentStrategy = paymentStrategy;
}
/// <summary>
/// Checkout method
/// </summary>
/// <param name="amount">Amout of cart checkout</param>
public void Checkout(double amount)
{
_paymentStrategy.Pay(amount);
}
}
The enchantment lay in the ability to switch between payment strategies seamlessly. Credit cards twirled with elegance, and PayPal pirouetted gracefully, all without a single modification to the core of the ShoppingCart class.
Act 3: The Symphony Unfolds
With the Strategy Pattern as his guide, John orchestrated a symphony of choices. The once rigid system now danced to the tune of flexibility, allowing for new payment methods to join the ensemble effortlessly.
CreditCardPayment creditCardPayment = new CreditCardPayment();
PayPalPayment paypalPayment = new PayPalPayment();
// Customer one is trying to pay by credit card.
ShoppingCart shoppingCartCustomer1 = new ShoppingCart(creditCardPayment);
shoppingCartCustomer1.Checkout(1000000); // This Customer is too rich :)
// Customer tow is trying to pay by Pay pal.
ShoppingCart shoppingCartCustomer2 = new ShoppingCart(paypalPayment);
shoppingCartCustomer2.Checkout(50); // This is me.
In this digital ballet, the beauty lies in the simplicity of choice. Developers could add new payment partners without disrupting the delicate choreography of the existing system.
Epilogue: The Ever-Adapting Strategy Design Pattern
And so, in this kingdom, the Strategy Pattern became a cherished tale told by developers around the virtual campfire. The moral of the story: in the face of evolving requirements, the Strategy Pattern stands as a beacon of adaptability, guiding developers through the ever-shifting landscape of software design.
nicely written as story